Type Module¶
The fundamental Ontic base data types for creation of derived child classes.
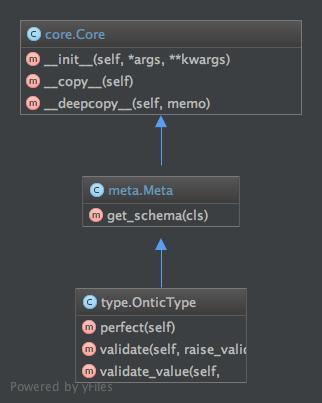
Contents
Usage¶
Create Ontic Types¶
The ontic_type module provides the OnticType
and a set of
functions to handle the creation and validation of OnticType instances.
Construction of Ontic data types as a class definition:
>>> class MyType(OnticType):
... ONTIC_SCHEMA = Schema({
... 'some_property': {
... 'type': 'int',
... 'required': True,
... },
... 'other_property': {
... 'type': 'str',
... 'required': False,
... 'enum': {'Enum1', 'Enum2', 'Enum3'}
... },
... })
>>> my_object = MyType()
>>> my_object.some_property = 7
>>> # or
>>> my_object['some_property'] = 7
>>> validate_object(my_object)
[]
Dynamic Ontic Type Definition¶
It is also possible to create OnticType
derived types dynamically
with the use of the create_ontic_type()
function.
>>> some_type = create_ontic_type('SomeType', {'prop':{'type':'int'}})
>>> my_object = some_type(prop=3)
>>> my_object
{'prop': 3}
>>> my_object.prop
3
Classes¶
OnticType¶
- class ontic.OnticType(*args, **kwargs)¶
OnticType provides the Ontic schema interface.
The OnticType provides the schema management functionality to a derived Ontic type instance.
- perfect() NoReturn ¶
Function to ensure complete attribute settings for a given object.
Perfecting an object instance will strip out any properties not defined in the corresponding object type. If there are any missing properties in the object, those properties will be added and set to the default value or None, if no default has been set.
For the collection types (dict, list, set), the default values are deep copied.
- Return type
None
- validate(raise_validation_exception: bool = True)¶
Validate the given OnticType instance against it’s defined schema.
- Parameters
raise_validation_exception – If True, then validate_object will throw a ValueException upon validation failure. If False, then a list of validation errors is returned. Defaults to True.
- Returns
If no validation errors are found, then None is returned. If validation fails, then a list of the errors is returned if the raise_validation_exception is set to True.
- validate_value(value_name: str, raise_validation_exception: bool = True)¶
Validate a target value of a given ontic object.
- Parameters
value_name – The value name to validate.
raise_validation_exception – If True, then validate_object will throw a ValueException upon validation failure. If False, then a list of validation errors is returned. Defaults to True.
- Returns
If no validation errors are found, then None is returned. If validation fails, then a list of the errors is returned if the raise_validation_exception is set to True.
Functions¶
create_ontic_type¶
- ontic.type.create_ontic_type(name: str, schema: (<class 'dict'>, <class 'ontic.schema.Schema'>)) ontic.type.OnticType ¶
Create an Ontic type to generate objects with a given schema.
create_ontic_type function creates an
OnticType
with a given name and schema definition. The schema definition can be a dict instance that is a valid schema definition or aontic.schema_type.SchemaType
. This makes the following forms valid:MyType = create_ontic_type('MyType', {'prop':{'type':'int'}}) schema_instance = Schema(prop={'type':'int'}) MyType = create_ontic_type('MyType', schema_instance)
perfect_object¶
- ontic.type.perfect_object(the_object: ontic.type.OnticType) NoReturn ¶
Function to ensure complete attribute settings for a given object.
Perfecting an object instance will strip out any properties not defined in the corresponding object type. If there are any missing properties in the object, those properties will be added and set to the default value or None, if no default has been set.
For the collection types (dict, list, set), the default values are deep copied.
- Parameters
the_object – Ab object instance that is to be perfected.
validate_object¶
- ontic.type.validate_object(the_object: ontic.type.OnticType, raise_validation_exception: bool = True)¶
Function that will validate if an object meets the schema requirements.
- Parameters
the_object (
OnticType
) – An object instant to be validity tested.raise_validation_exception – If True, then validate_object will throw a ValueException upon validation failure. If False, then a list of validation errors is returned. Defaults to True.
- Returns
If no validation errors are found, then None is returned. If validation fails, then a list of the errors is returned if the raise_validation_exception is set to True.
- Raises
ValueError – If the_object is None or not of type
OnticType
.ValidationException – A property of the_object does not meet schema requirements.
validate_value¶
- ontic.type.validate_value(property_name: str, ontic_object: ontic.type.OnticType, raise_validation_exception: bool = False)¶
Validate a specific value of a given
OnticType
instance.- Parameters
property_name – The value to be validated against the given PropertyType.
ontic_object – Ontic defined object to be validated.
raise_validation_exception – If True, then validate_object will throw a ValueException upon validation failure. If False, then a list of validation errors is returned. Defaults to True.
- Returns
If no validation errors are found, then None is returned. If validation fails, then a list of the errors is returned if the raise_validation_exception is set to True.
- Raises
ValueError – If property_name is not provided or is not a valid string.
ValueError – If ontic_object is None, or not instance of OnticType.
ValidationException – If the validation is not successful. The ValidationException will not be raised if raise_validation_exception is set to False.